In recent years, JavaScript has become the foundation for a rapidly evolving landscape of Frameworks and Libraries. There seems to be a new kid on the block every few weeks or so, and with this consistent influx of new and innovative tools, it can be difficult to know what will stick, and what won’t survive it’s first birthday. A few years ago, we began using AngularJS as the framework of choice for some of our larger web apps. Ever since then, I’ve begun phasing things like jQuery out of our projects wherever possible in favor of AngularJs or pure JavaScript.
Through the course of this article, I’m going to go over a small comparison between jQuery and AngularJS and why here at Brolik we choose to use one over the other. We’ll start by looking at some of the functional differences between the two, then at the difference in performance when simply loading the two, and finally a small example project.
Before we begin, I want to preface this by saying comparing AngularJS to jQuery is a bit like comparing apples to oranges. They’re similar enough that the comparison is worthwhile to make, but they’re generally used for two different things.
Comparison
Ajax / JSONP - First, what are they? Ajax stands for Asynchronous JavaScript + XML, though JSON is used far more often than XML these days. JSONP is JSON with Padding. Both of these are a way to transfer data between the client and another server, without refreshing the page. Both AngularJS and jQuery provide easy access to this feature, AngularJS through its `$http` module, and jQuery through the `$.ajax()` function.
Animation Support - Both systems allow for the use of animation. AngularJS requires an additional library (ngAnimate), where as jQuery has direct access to the .animate() function. My advice, just use CSS for your animation. Animation is flashy and cool but who cares if it doesn’t work in IE9?
Template Support - This is where we begin to see some differences between the two. Without the use of ES6 or Babble, it's difficult to add templates into jQuery. How many times have you written something like this?
<span style="font-weight:400;">$(‘.element’).click(function () {</span> <span style="font-weight:400;"> $(this).find(‘.child’).append(‘<div class=”form-row”> +</span> <span style="font-weight:400;"> ‘<input type=”text” name=”list[]” />’ +</span> <span style="font-weight:400;"> ‘</div>’</span> <span style="font-weight:400;"> );</span> <span style="font-weight:400;">}); </span>
There's nothing wrong with that, and it works, but it doesn’t syntax highlight. And what happens if you’re using that same bit of HTML in multiple locations? It makes it very difficult to keep your code DRY (Don’t Repeat Yourself).
AngularJS on the other hand, allows you to set directives and templates to control your layout a little bit easier, even if it might be more code. The primary difference is that AngularJS’s templates are called in a mix of HTML, and JavaScript:
Page.html
<span style="font-weight:400;"><div template-name></div></span>
Template-name.html
<span style="font-weight:400;"><div class=”template”></span> <span style="font-weight:400;"> <div class=”form-row”></span> <span style="font-weight:400;"> <input type=”text” name=”list[]” ng-model=”list[]”></span> <span style="font-weight:400;"> </div></span> <span style="font-weight:400;"></div></span>
Template-name.directive.js
<span style="font-weight:400;">‘use strict’;</span> <span style="font-weight:400;">angular.module(‘myDirective’, []).directive(‘templateName’, [function () {</span> <span style="font-weight:400;"> return {</span> <span style="font-weight:400;"> restrict: ‘A’,</span> <span style="font-weight:400;"> templateUrl: ‘template-name.html’</span> <span style="font-weight:400;"> }</span> <span style="font-weight:400;">}]); </span>
While AngularJS requires three files to create a template in this instance, it helps to keep your code more modular and organized throughout an entire project.Keep in mind this isn’t the greatest example of a template, generally they’re more complicated than a single input.
Dependency Injection
This isn’t a necessity in jQuery. As long as you don’t have your scripts called as anonymous functions you can generally access your plugins no problem. But you don’t always need to load that library on every page. Dependency injection is primarily used for modularity in a project, (again keeping it Dry) and you can accomplish something similar with JavaScript objects. It’s all about keeping your functions local so that they run in a controlled environment.
AngularJS uses heavy Dependency Injection. In fact, everything about AngularJS needs to be injected. If you want to use the `$http` request, you need to inject it into your controller:
<span style="font-weight:400;">.controller(‘myController’, [‘$http’, function ($http) {</span> <span style="font-weight:400;"> // Now you can use $http</span> <span style="font-weight:400;">}]); </span>
Two Way Data Binding
Now we get into the good stuff with AngularJS. Two-way data binding is both a blessing and potential curse. It allows for direct modification of the DOM and on screen html as the user types and interacts with your site. jQuery can kind of do this, but it requires you to write JavaScript to do so, as opposed to AngularJS, where it’s built in directly.
Why is this good? Well, the easiest example is that with AngularJS it allows you to do fuzzy searching and filtering as the user types.
Angular:
See the Pen yoQMVN by Kyle Jasso (@jassok) on CodePen.
In this example, we have a simple input, and an output, to display what two way databinding does. Then, a real world use case, where you can filter a user list in a table. With angular, the only javascript we’re required to write is the array that contains the user list.
jQuery:
See the Pen ZJmeML by Kyle Jasso (@jassok) on CodePen.
The jQuery example, has the same functionality that the angular version does, with the main difference being that there’s 50 lines of javascript written to do the same functionality. The filtering, and data binding should be written to be more modular across the application if it's something you actually wanted to use in production.
Form Validation
This is another one of those things that AngularJS comes with out of the box. Adding a form element into your page gives you access to several functions in the DOM. The following being some of the most useful:
<span style="font-weight:400;">$scope.formName.$dirty: boolean</span>
True if the user has already interacted with the form.
<span style="font-weight:400;">$scope.formName.$invalid: boolean</span>
True if the use is missing required fields.
<span style="font-weight:400;">$scope.formName.$pristine: boolean</span>
True if the user has not interacted with the form yet.
jQuery, on the other hand, doesn’t offer any of these features. You can write them yourself, or use a third party plugin such as the jQuery Validation Plugin.
There are plenty of other differences between AngularJS and jQuery, but these are just a few of the most common things I run across while working on a project. (Often times wishing jQuery would just do two-way data binding, or easier form validation.)
Performance and File size
Outside of the difference in features, performance and file size are very important things to consider when picking a JavaScript framework or library to use. People have a tendency to forget phones, or take for granted data limits and usage, so I like to err on the side of performance. If the site loads quickly, size generally isn't an issue.
For this, we’re going to look at the project size and load times of an AngularJS project and a jQuery project that load their respective libraries from the folder and a CDN (Content Delivery Network).
File Size
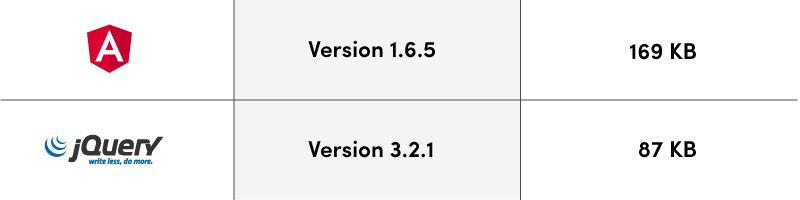
At the time of writing, the minified version of AngularJS is nearly double that of jQuery.
Performance
For these numbers, we’re looking at the average load time of a very simple page that simply includes the minified AngularJS or jQuery file, and runs a `console.log` in the window. These numbers are averaged over 10 loads each.
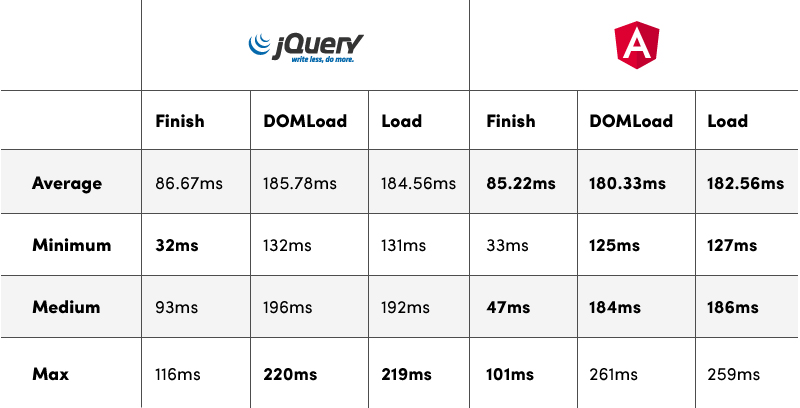
First thing to note about this, is that it’s almost never a good idea to include libraries directly from your source, but that's for another discussion about CDNs. However, based off these numbers, we can see that even with a larger file size, AngularJS had a better average load time, DOMLoad, and finish.
Next, we’ll take a look at loading the same project, only this time including the library from the CDN provided directly from the websites where you can download each. You can obviously include each from multiple different CDNs to find one that is quicker if you need to.
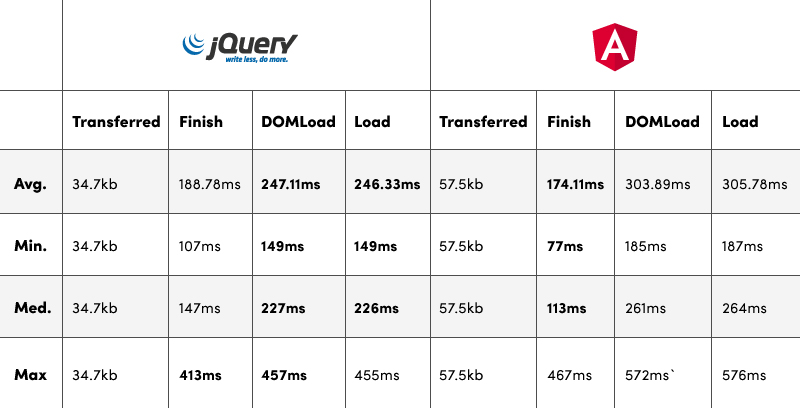
When loading from the CDN, we see a slightly different story. AngularJS finishes sooner, but jQuery loads the DOM faster.
- DOMContentLoaded (DOMLoad is an event fired when the initial HTML document is completely loaded and parsed without stylesheets, images, and subframes loaded).
- Load is fired when a resource and all its dependencies have finished loading.
- Finish, is the time it takes to load asynchronous content, or minimum requirements for something to show up on the page.
Example Project
I mentioned in the beginning that there's a new JavaScript framework out every other week, and TodoMVC is a great resource to compare and contrast these different frameworks. It's a very simple app, that allows a user to add, edit, delete, and complete tasks. We’re going to use this as the comparison example.
First up, let's look at some stats about the different projects.
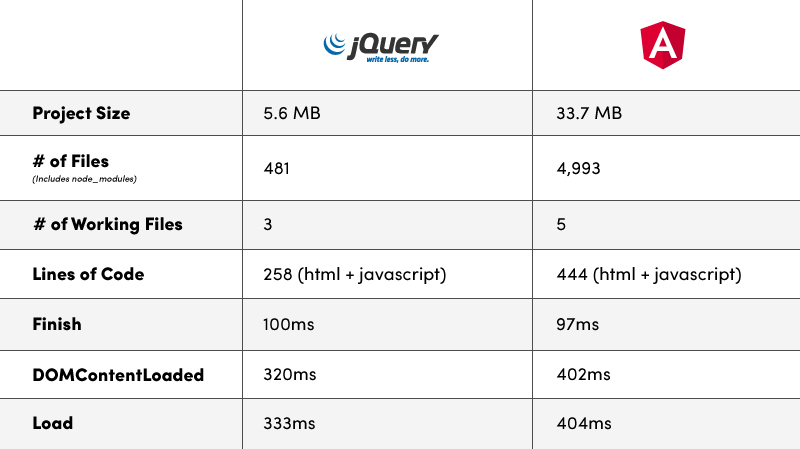
You can find these examples and more on TodoMVC: AngularJS - jQuery
An important note about the size of the two projects is that the AngularJS project has testing in it (Karma and Jasmine). The angular project also has more working files to pay attention to. Load times are very similar as well.
The projects are very small, and it shouldn’t take long to review the different style of code between AngularJS and jQuery.
Which one should you use?
As initially mentioned, comparing AngularJS to jQuery is a bit like comparing apples to oranges. AngularJS is a framework, and jQuery is a library. Both offer a decent amount of functionality, the performance is similar, and size difference is negligible.
The general distinction that I tend to make involves how much interaction is needed from the user. jQuery falls short with ease of use and organization when there’s a large amount of data, forms, and rapidly changing content. That's not to say that you couldn’t do everything with jQuery that you can with AngularJS, but for me, I find it quite telling that the jQuery library is half the size of AngularJs and yet it loads slower. This is just a personal opinion, but I feel that jQuery is fairly bloated and could be due for some optimization.
As I work more with JavaScript, I find myself discovering more and more reasons to avoid jQuery in favor of pure JavaScript whenever possible. However, it is still an excellent choice when you need minimal JavaScript interaction.
AngularJS on the other hand, is excellent for large scale or interactive projects. It offers a lot of really useful built-in features that I haven’t touched on, such as the power of `ng-repeat`, directives, and services that all help you to keep your application or website DRY. As with most tools, it has its own drawbacks and can be overkill in some situations.
So what should you use? Well, a bit of it is personal preference. I personally don’t like using jQuery, and for me and the other Developers here at Brolik it's a last resort fall back. We’ve been making an effort to improve the optimization of our websites, and jQuery tends to make this more difficult for us to do so. For me then, the answer is AngularJS, but that's down to a personal liking of the framework.